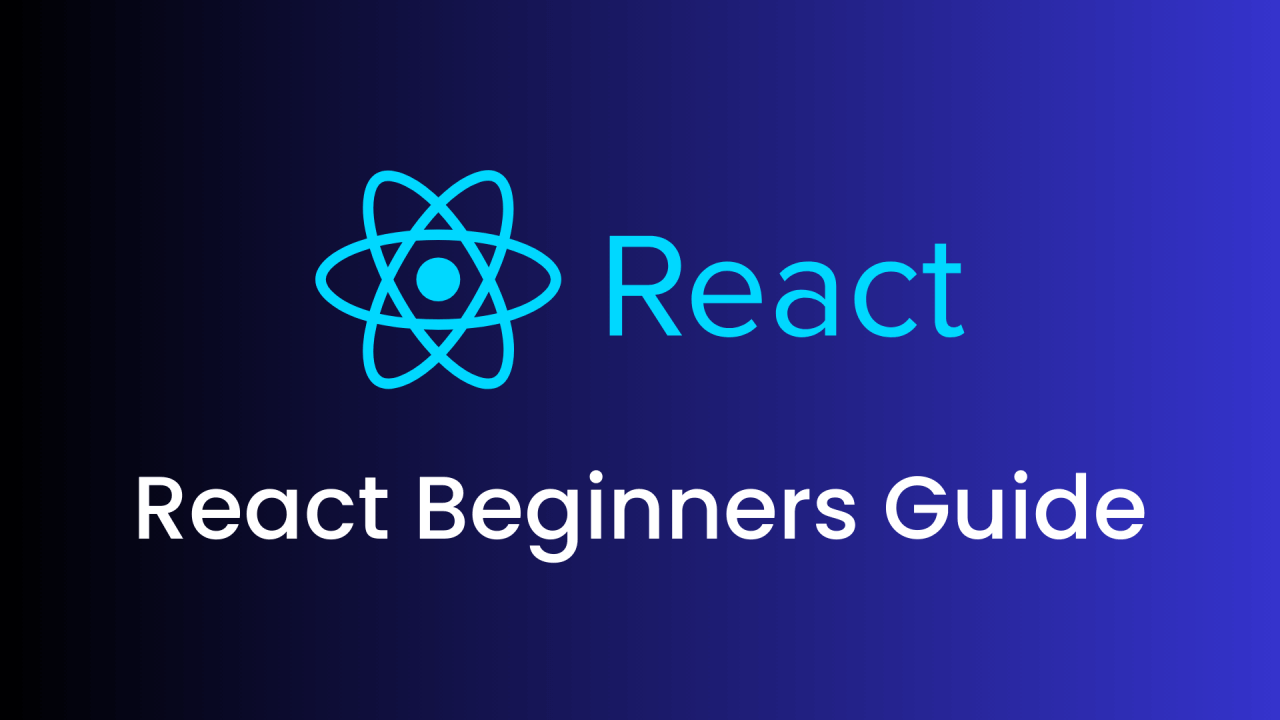
Go Back
Beginner's Guide to React: Getting Started with Building Dynamic Web Apps
Mar 13, 2024 • 5 min
What is React?
React is a popular JavaScript library used for building user interfaces, particularly for single-page applications where data can change over time. Developed and maintained by Facebook, React has gained widespread adoption due to its efficiency and flexibility.
Prerequisites
Before diving into React, make sure you have a basic understanding of:
• HTML
• CSS
• JavaScript (ES6+)
• HTML
• CSS
• JavaScript (ES6+)
Setting Up Your Environment
To start using React, you'll need to set up your development environment:
1. Node.js and npm: React requires Node.js and npm (Node Package Manager) to manage dependencies and run scripts.
2. Create React App: This is the easiest way to start a new React project. Install it globally using npm:
1. Node.js and npm: React requires Node.js and npm (Node Package Manager) to manage dependencies and run scripts.
2. Create React App: This is the easiest way to start a new React project. Install it globally using npm:
npm install -g create-react-app
Creating Your First React App
Once you have create-react-app installed, you can create a new React project:
1. Open your terminal or command prompt.
2. Navigate to the directory where you want to create your project.
3. Run the following command to create a new React app named my-react-app:
1. Open your terminal or command prompt.
2. Navigate to the directory where you want to create your project.
3. Run the following command to create a new React app named my-react-app:
npx create-react-app my-react-app
Understanding the Folder Structure
A typical React project generated by create-react-app will have the following structure:
• node_modules/: Contains all the project dependencies.
• public/: Contains the static assets like HTML files and images.
• src/: Contains the source code for your React application.
• node_modules/: Contains all the project dependencies.
• public/: Contains the static assets like HTML files and images.
• src/: Contains the source code for your React application.
• index.js: Entry point of the application.
• App.js: Main component where you'll start building your app.
• App.css: CSS styles for the App component.
• App.js: Main component where you'll start building your app.
• App.css: CSS styles for the App component.
npm install -g create-react-app
Writing Your First Component
In React, everything revolves around components. A component is a JavaScript function or class that returns a piece of JSX (JavaScript XML) which represents the UI.
1. Open src/App.js.
2. Replace the contents with the following code to create a simple component:
1. Open src/App.js.
2. Replace the contents with the following code to create a simple component:
import React from 'react';
// Example: Creating a simple button component
function MyButton() {
return (
<button>
I'm a button
</button>
);
}
export default App;
Running Your React App
After creating your component, you can run your React app locally:
1. Navigate to your project directory my-react-app.
2. Run npm start in the terminal. This will start the development server and open your app in the browser.
1. Navigate to your project directory my-react-app.
2. Run npm start in the terminal. This will start the development server and open your app in the browser.
Learning More
Now that you've created and run your first React app, here are some next steps to continue learning:
• JSX: Dive deeper into JSX syntax and how it integrates JavaScript with HTML.
• Components: Learn about functional and class components, state, and props.
• React Router: Explore how to add routing to navigate between different pages.
• State Management: Understand how to manage state with React hooks or Redux.
• API Integration: Learn how to fetch data from APIs and display it in your app.
• JSX: Dive deeper into JSX syntax and how it integrates JavaScript with HTML.
• Components: Learn about functional and class components, state, and props.
• React Router: Explore how to add routing to navigate between different pages.
• State Management: Understand how to manage state with React hooks or Redux.
• API Integration: Learn how to fetch data from APIs and display it in your app.
Conclusion
React provides a powerful way to build interactive user interfaces for web applications. By understanding the basics covered in this guide and continuing to explore its ecosystem, you'll be well on your way to mastering React development.